You’ve got a backstage pass to PUSSY SUBLIMITY! A band composed of me, Eden Chinn, and Eric Chai! Our method is to use performance to explore sublimity and all things fine and feline.
Our first project for Physical Computing was to construct a simple Single-note Katzenklavier! The Katzenklavier, or cat organ, was “an instrument played by pressing keys attached to nails, which in turn strike the tails of a series of cats arranged with care so as to miaow in tonally sequenced pain.”
The experience of playing this instrument was intended to be so absurd that it would “humble” the player and help ground their rational selves/ego.
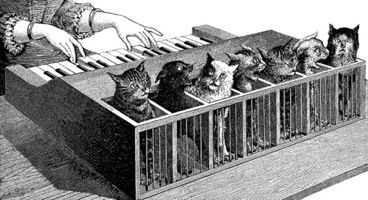
image description: an illustration of the Katzenklavier, an organ comprised of piano keys and cats. The image depicts the katzenklavier with two and a half octaves worth of piano keys. Attached to the organ, are seven cages, each cage containing a cat. A pair of hands are delicately playing the keys on the organ and the cats that correspond to the keys pressed are meowing in pain.
For the scope of the project, we decided to keep it to a single piano key/button. Our plan was to have our katzenklavier emit the recording of a ‘meow’ every time the piano key was pressed. We wanted to have the katzenklavier play the full recording, even if the piano key was released and we wanted the recording to loop if the piano key remains pressed.
Building our own Katzenklavier would require several things: a functioning circuit, working code, and cardboard housing.
A FUNCTIONING CIRCUIT:
We started constructing our circuit by drafting a schematic diagram! I included the explanation of this circuit in the image description below.
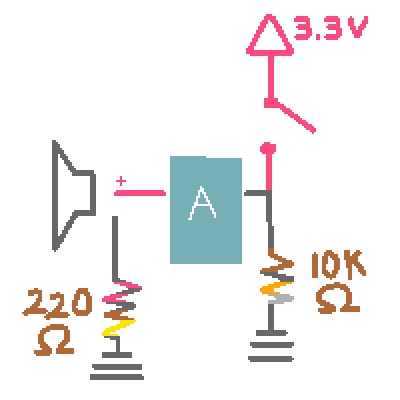
Image Description: a schematic diagram for our Katzenklavier. This schematic features an Arduino, a speaker, two resistors, and a switch.
The circuit is powered by 3.3 volts, which is depicted in the upper right corner of the image. A switch, which represents our ‘piano key’, is shown next in the circuit, to determine the flow of the current.
If the key is unpressed, the current will run through the 10k resistor, which will “pull down” to ground. This “pulling down” ensures the avoidance of any floating electrical current and that our code will read the switch correctly.
If the piano key is pressed, the switch directs the current to our Arduino, which is programmed to provide power to our speaker to emit our audio file. After running through our speaker, the current will then flow to our 220 ohm resistor, and then to ground.
I assembled the breadboard accordingly! We chose to connect the speaker to the DAC pin (pin 14) on the Arduino for the cleanest sound. The D8 pin was chosen for our input because it looked like a screaming face, which perfectly embodied our exploration of sublimity.
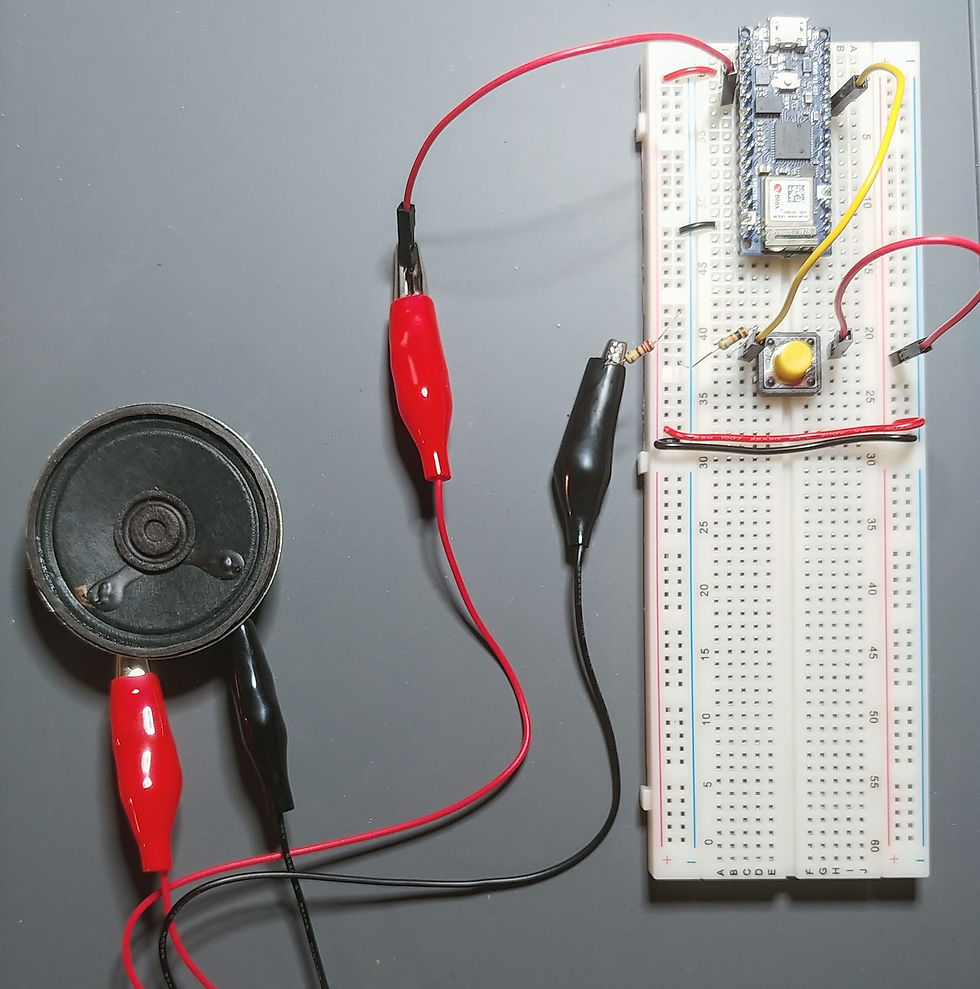
Image description: A picture of a breadboard which features an arduino, a speaker, two resistors, and a switch. The speaker is positioned to the left of the breadboard, and is connected to the fourth pin down on the left side of the Arduino by a red wire. A yellow wire, which represents our input, is connected to the fifth pin down on the right side of the Arduino and the top left pin of a yellow pushbutton, placed underneath the Arduino in the middle of the breadboard. A red power, which connects the pushbutton to power is connected to the top right pin of the yellow pushbutton.
Unfortunately, the circuit didn’t work at first! After some quick tinkering, we realized that it was because the wire that was connecting the switch to power should be on a separate row than the button’s pin which was connected to the resistor.
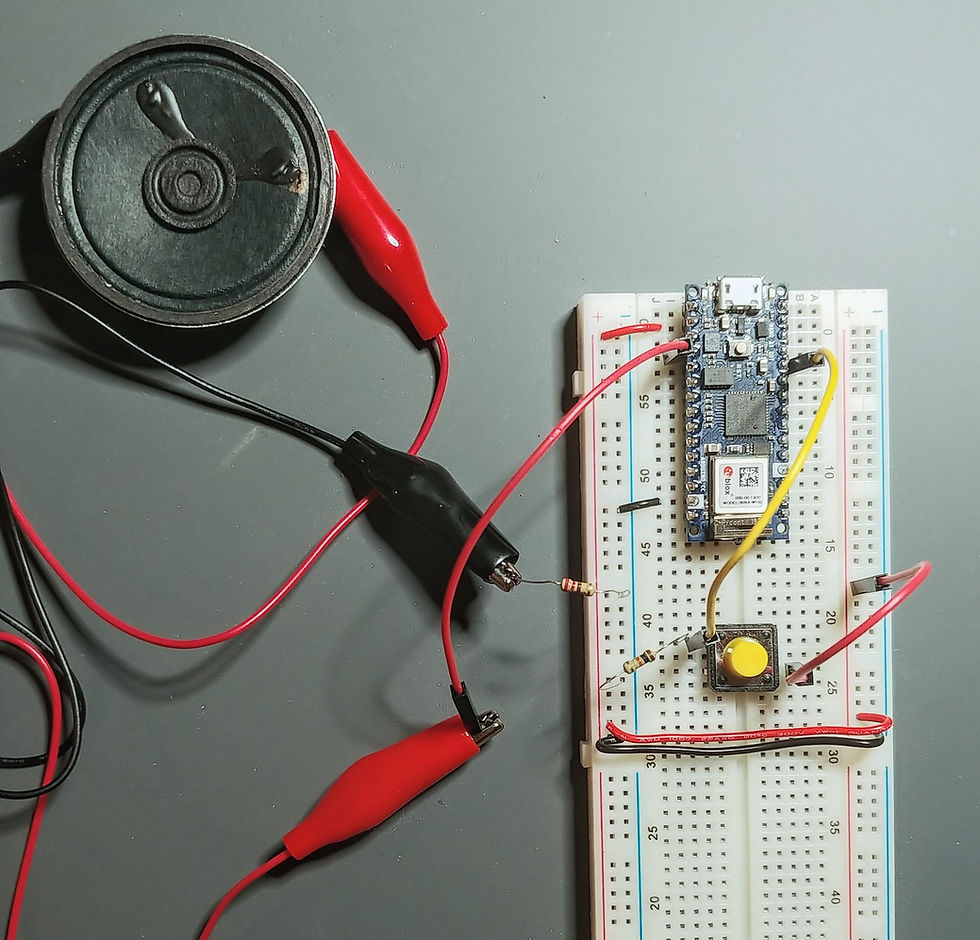
Image description: A picture of a breadboard which features an Arduino, a speaker, two resistors, and a switch. This breadboard features the same connections as the previous image, but the red wire which connected the pushbutton to power has been moved. It now is connected to the bottom right pin of the pushbutton.
HOORAY!! This gets us to our next step,,,
WORKING CODE:
Our goal was to have our single-note katzenklavier play a recording. However, to just test the circuit to see if it worked, we just had the speaker emit a simple tone. Our first draft of our code read like this (comments are in blue):
void setup() {
// pin for input is D8, which is 5 down on the right side of the arduino, we're using this because it looks like a terrified face (how sublime)
// pin for output is the dac0 , pin D14 which is 4 down on the left side of the arduino
pinMode(8, INPUT); //this is to set up our piano key
pinMode(14, OUTPUT); // this is for our speaker
}
void loop() {
// put your main code here, to run repeatedly:
// read the piano key input
if (digitalRead (8) == HIGH) { //HIGH refers to if the voltage is greater than a specific amount, this amount is dependent on the input or output
// if the piano key is pressed
tone (14, 440, 1000);
//TONE PLAY FOR 1 SECOND
delay(1000); // this is so the arduino can read the code
}
else {
// if the switch is unpressed
noTone(14);
//don't play anything shhhhh
}
}
This worked wonderfully with our functioning circuit! The next step was to have the code play a specific recording. To do so, we heavily referenced this High-low tech tutorial (SHOUTOUT TO BRANDON ROOTS, FELLOW CLASSMATE AND COMRADE WHO SHARED THIS LINK WITH ME, UR THE MVP, BRANDON).
This process required translating our audio files into a 2-dimensional string of numbers which the Arduino could then relay to our speaker. We learned a lot about how this process worked from our fellow bandmate, Eric Chai!
A QUICK BREAK FROM MAKING, IT'S TIME TO LEARN!!!
Microphones and speakers are transducers, meaning that they have the ability to convert one form of energy to another! Microphones translate pressure waves to electrosignals, and speakers work the other way around.
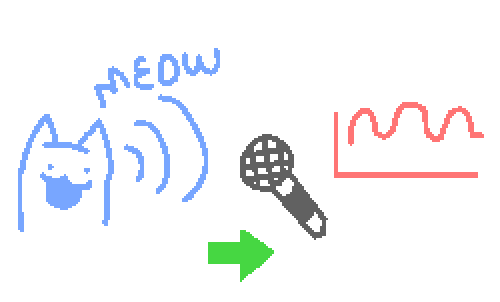
Image description: An illustration of a cat's 'meow' being converted by a microphone to an electrosignal. This picture is very nice.
Of course, there are limitations to being able to record pressure waves perfectly! There are limitations within the hardware that prevent us from being able to attain the extensive amount of data for a perfect recording. Instead, the transducer samples the pressure wave within thousandths of second intervals. The smooth, wavy form of a sound wave is translated into convenient blocks of data.
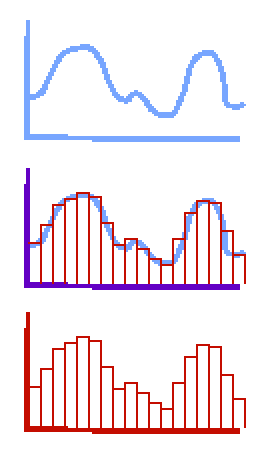
Image description: An illustration of the process of translating a pressure/sound wave to electrosignal. There are three different graphs, each graph representing one step in the process.
The first graph depicts the original pressure wave, which is wavy and curvy. The pressure wave is drawn in blue line.
The second graph depicts the sampling of the original pressure wave. Several vertical lines are imposed over the wave, and represent the thousandths of second intervals in which the wave is sampled. Horizontal lines average out the height, or y values of the pressure wave. The lines that depict sampling are rendered in red.
The last graph depicts the final sampling of the original blue, pressure wave. It is a series of vertical blocks that match the height of the original pressure wave.
There is a way for this data to be represented through a set of numbers, each number describing the height and sample rate of the sound file. Thankfully, the High-Low tech tutorial provided a tool that converted our audio files to this series of values. Even though our audio files were only a second long, and 10-bit resolution, it resulted in hundreds of lines of numerical values. Eric helped us convert all of it into a convenient header file, which we could then include in our code.
In order to use the header file in our code, we had to make sure that it was in the same location as our Arduino code! We also included this bit of text at the very top of our code (before void setup) to reference our file!
#include "meow.h"
(meow.h is the name of our header file)
Our entire code looks like this:
#include "meow.h"
void setup() {
// pin for input is D8, which is 5 down on the right side of the arduino, we're using this because it looks like a terrified face (how sublime)
// pin for output is the dac0 , pin D14 which is 4 down on the left side of the arduino
pinMode(8, INPUT); //this is to set up our piano key
pinMode(14, OUTPUT); // this is for our speaker
analogWriteResolution(10); // this makes the sound more clear
}
void loop() {
// this is to define our audio sample stuff
long i = 0;
int usec_per_sample = 1000000 / rate; // this is the sample rate!
// read the piano key input
if (digitalRead (8) == HIGH) {
// if the piano key is pressed
while (true) {
if (i >= num_samples) // length of the file, when it reaches the end of the file, play it over (this plays the role of the delay that we had in our previous rendition of code)
break; // this allows the audio to stop looping when the key is not pressed
analogWrite(14, samples[i]); // this tells the arduino to play the audio file on our speaker
unsigned long start = micros();
while (micros() - start < usec_per_sample); // it takes current time in microseconds, and compares current time to its start, once it reaches a certain amount of miliseconds, then its done
i++; // we reached the end of sample, move to next sample
}
}
else {
// if the switch is unpressed
noTone(14);
//cat is asleep shhhhh
}
}
CHECK OUT THAT MEOW
THE CARDBOARD HOUSING
Any proper cat-inspired project should acknowledge the existence of cardboard. I was very excited to construct the external housing that would host our electric components. I drafted a couple of sketches.

The image on the left is an illustration of the insides of the single-note katzenklavier, the image on the right shows it fully assembled with all of its components. We plan to upload a pre-recorded “miaow” sound, which will be played through the speaker when the piano key is pressed.
On the diagram on the left, you can see several electronic components arranged in a cardboard tray. There is a switch/push button in yellow, which will communicate with our microcontroller, which is illustrated as a teal rectangular prism. The last component is a speaker, which is mounted at the far end of the cardboard tray.
The right diagram features the fully assembled instrument, including several cardboard components which are intended to make our device look more like a cat organ! There is a simple cardboard piano key which fits into a rectangular-shaped hole cut into the cardboard frame of the device. Covering the speaker in our device is a cardboard cat head, which will also act as a funnel to help project sound.
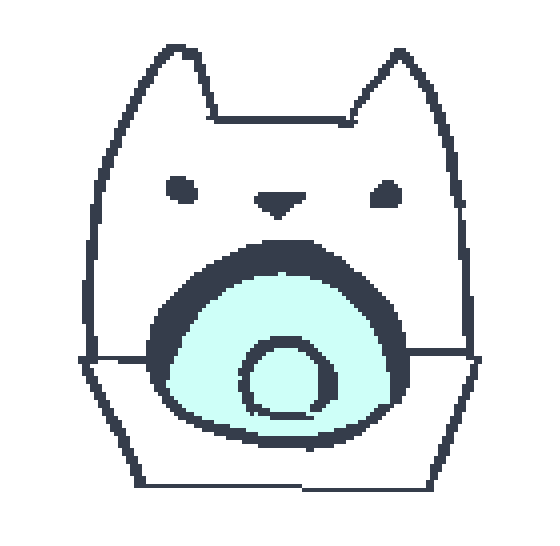
This illustration is intended to be the front of the device! You can see the seam between the bottom tray, which houses our electronic components, and our top external covering. A speaker, which is coloured light blue, is housed neatly within the external cardboard housing.
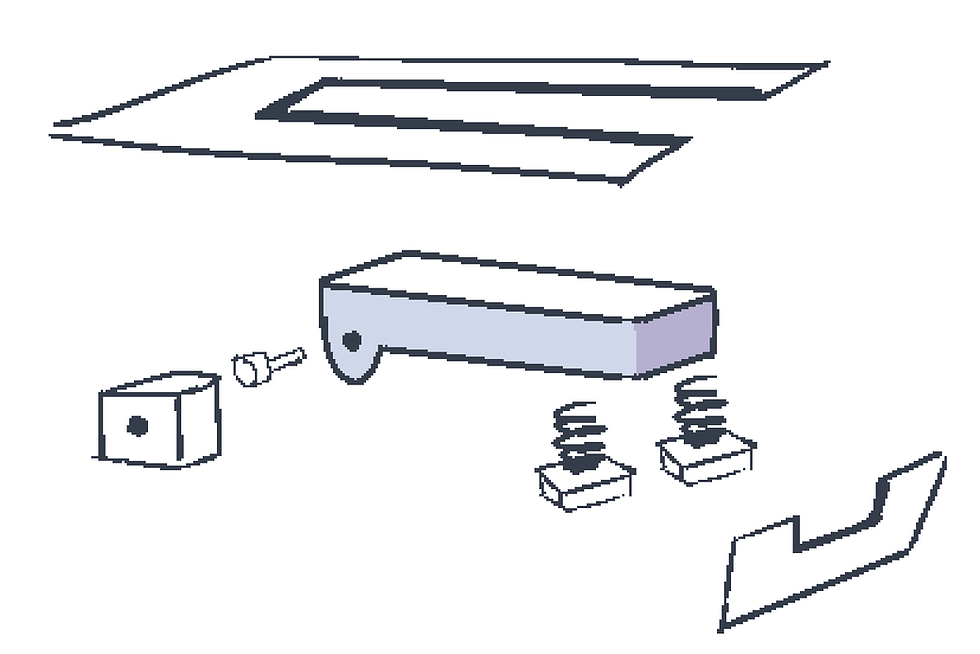
This illustration depicts the various mechanical components for our cardboard piano key. There is a hinge featured on the end of the piano key, which will be connected to cardboard on the side. Two springs will be used underneath the key to keep it rested in an “unpressed” position. There are two pieces of cardboard illustrated on the sides, which have holes to make room for the key. When the key is pressed, it will compress the springs and make contact with the pushbutton, placed on the breadboard.
I spent a good bit of time measuring and constructing the cardboard housing. The original plan for my push button ended up not working. The springs that I ordered ended up being too big, so I just had the cardboard piano key sit directly on top of the pushbutton.
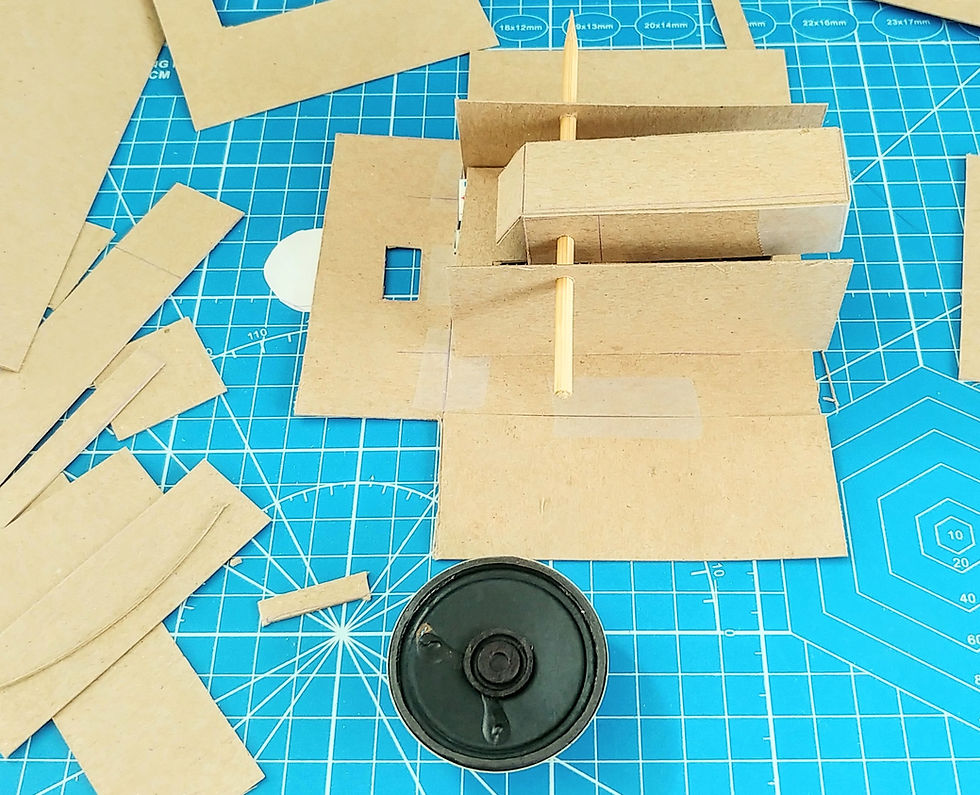
Image description: A photograph of my workstation. Various scraps of cardboard are scattered to the left of the image. Featured in the center of the photograph is a cardboard mechanism intended to emulate a piano key. A long, rectangular prism, which represents the piano key, is suspended by a wooden skewer which is mounted on adjacent cardboard structures which run parallel to the piano key. In the very front of the image is a speaker, placed for scale. The bottom of the cardboard tray is around 14 cm across.
I really wanted to be able to access the electronic parts without completely taking the katzenklavier apart. I decided to have the breadboard rest on the bottom tray, and for the piano key to be attached to the "lid" of the katzenklavier, and be completely detachable from the bottom tray.

Image description: A photograph of my workstation. There are two man components of cardboard, a bottom tray and a lid. The bottom tray features a breadboard, which has several wires and a pushbutton attached to it. The top lid features a piano key. A speaker is also placed at the bottom right corner of the photograph.
Here is a photograph of the cardboard housing fully disassembled.
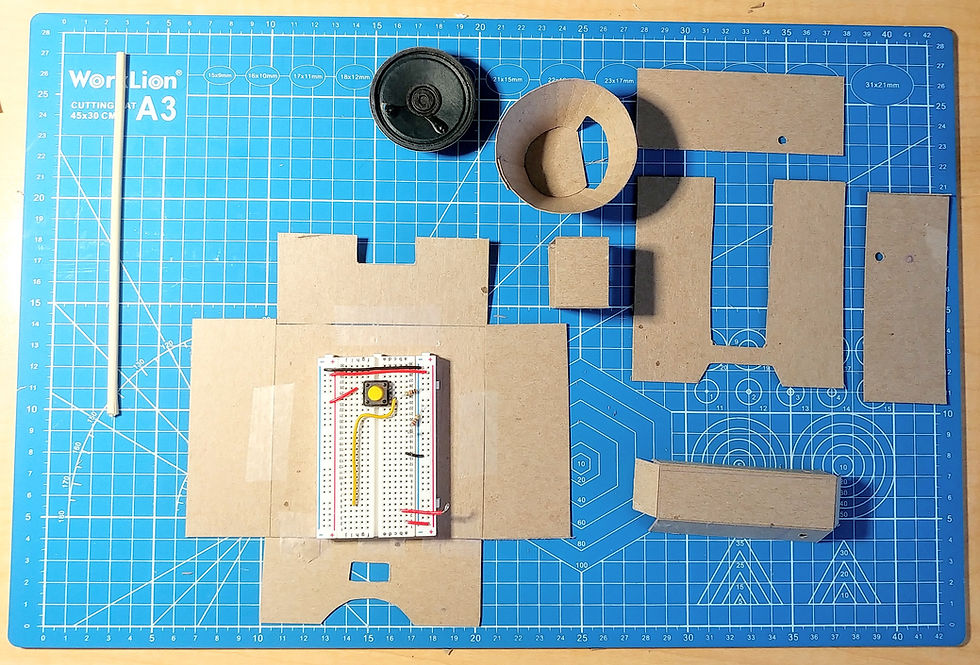
Image description: A photograph of the fully disassembled cardboard housing. Various faces of the bottom tray and the lid have been cut apart. Featured on top of the disassembled cardboard tray is a breadboard. A funnel designed for a speaker is featured at the top of the image.
I traced the cardboard pieces once I was pleased with the housing so that me and my collaborators could reference the template.
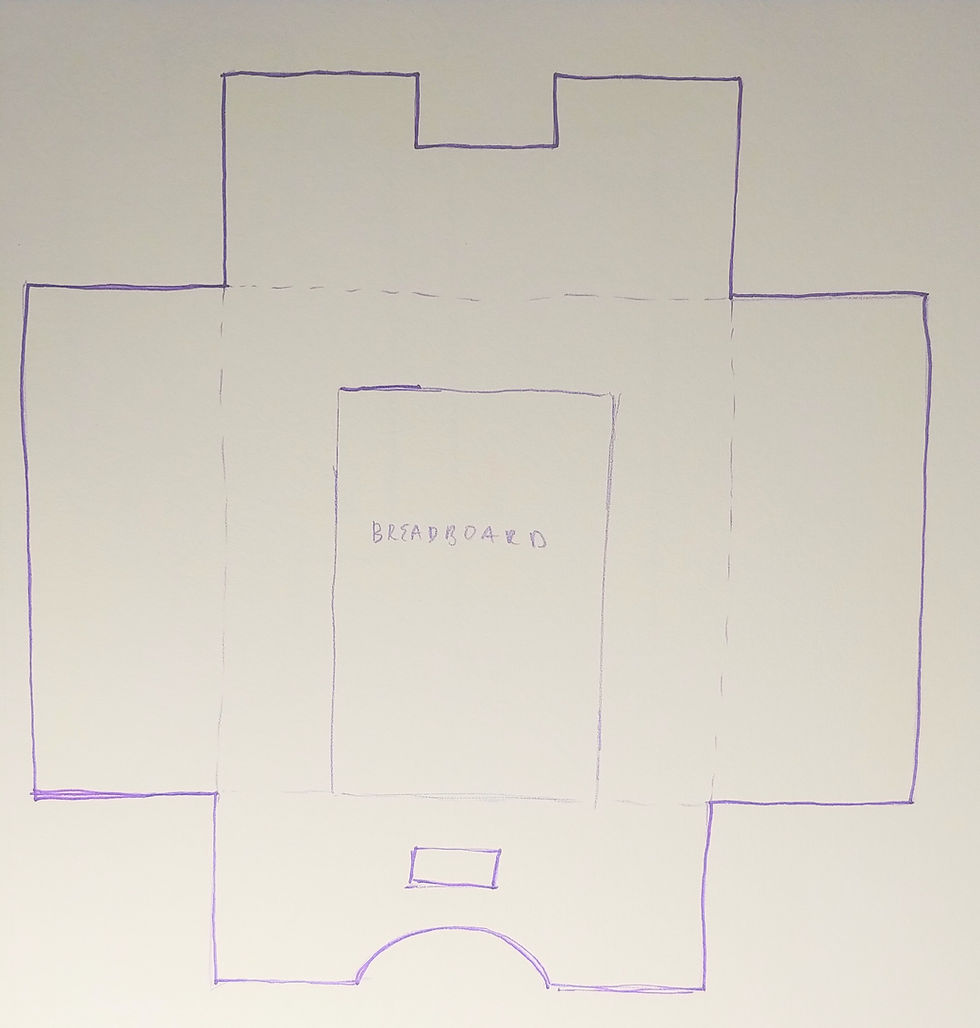
Image description: A photograph of the template that I traced for the bottom tray. There is a designated space for the breadboard.
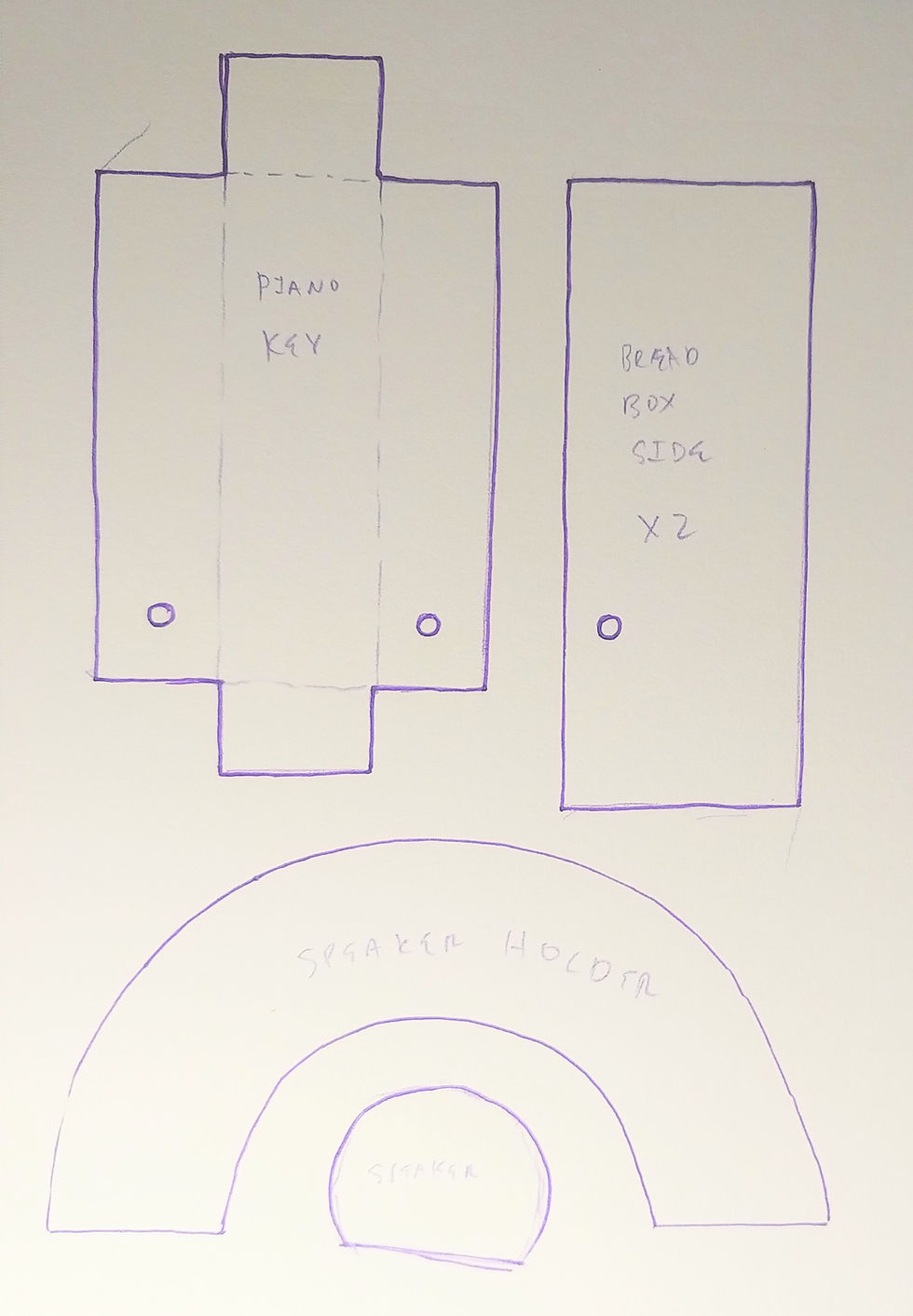
Image description: A photograph of the template that I traced for the piano key, lid sides, and speaker.
HOORAY HOORAY!!! All of the cardboard bits and decoration which will make this box more cat-like will come later. BUT FOR NOW SHE'S DONE!!! THE SINGLE NOTE KATZENKLAVIER!!!!

Image description: A photograph of the fully assembled katzenklavier. A small, cardboard box with a cardboard piano key and a speaker protruding from its side.
Comments